Slot Machine In Javascript
Shuffle your elements, randomize your results or make an slide. JQuery-SlotMachine is not a simple animation plugin. My question begins here. Ive been searching for a javascript code to make a small game for my site. It consists of a slot machine with a variety of rewards. Ive found a code which ive altered slightly however i cannot work out how to edit certain parts. For example here is the code.
Slot Machine In Javascript Programming
Math
Math
is one of JavaScript's global or standard built-in objects, and can be used anywhere you can use JavaScript. It contains useful constants like π and Euler’s constant and functions such as floor()
, round()
, and ceil()
.
Slot Machine with Fruit theme. The game starts with 5000 credits, and you can bet 30, 60 or 90 credits. Click on the bet buttons to make a bet, and then press the 'Spin' button. The game calculates the winnings based on the bet level, the configuration of the matching pattern,. An application of math.floor: How to Create a JavaScript Slot Machine For this exercise, we have to generate three random numbers using a specific formula and not the general one. Math.floor (Math.random. (3 - 1 + 1)) + 1.
In this article, we'll look at examples of many of those functions. But first, let's learn more about the Math
object.
Example
The following example shows how to use the Math
object to write a function that calculates the area of a circle:
Math Max
Math.max()
is a function that returns the largest value from a list of numeric values passed as parameters. If a non-numeric value is passed as a parameter, Math.max()
will return NaN
.
An array of numeric values can be passed as a single parameter to Math.max()
using either spread (..)
or apply
. Either of these methods can, however, fail when the amount of array values gets too high.
Syntax
Parameters
Numbers, or limited array of numbers.
Return Value
The greatest of given numeric values, or NaN
if any given value is non-numeric.
Examples
Numbers As Parameters
Invalid Parameter
Array As Parameter, Using Spread(…)
Array As Parameter, Using Apply
Math Min
The Math.min() function returns the smallest of zero or more numbers.
You can pass it any number of arguments.
Math PI
Math.PI
is a static property of the Math object and is defined as the ratio of a circle’s circumference to its diameter. Pi is approximately 3.14149, and is often represented by the Greek letter π.
Examples
More Information:
Math Pow
Math.pow()
returns the value of a number to the power of another number.
Syntax
Math.pow(base, exponent)
, where base
is the base number and exponent
is the number by which to raise the base
.
pow()
is a static method of Math
, therefore it is always called as Math.pow()
rather than as a method on another object.
Examples
Math Sqrt
The function Math.sqrt()
returns the square root of a number.
Java Slot Machine Program
If a negative number is entered, NaN
is returned.
sqrt()
is a static method of Math
, therefore it is always called as Math.sqrt()
rather than as a method on another object.
Syntax
Math.sqrt(x)
, where x
is a number.
Examples
Math Trunc
Math.trunc()
is a method of the Math standard object that returns only the integer part of a given number by simply removing fractional units. This results in an overall rounding towards zero. Any input that is not a number will result in an output of NaN.
Careful: This method is an ECMAScript 2015 (ES6) feature and thus is not supported by older browsers.
Examples
Math Ceil
The Math.ceil()
is a method of the Math standard object that rounds a given number upwards to the next integer. Take note that for negative numbers this means that the number will get rounded “towards 0” instead of the number of greater absolute value (see examples).
Examples
Math Floor
Math.floor()
is a method of the Math standard object that rounds a given number downwards to the next integer. Take note that for negative numbers this means that the number will get rounded “away from 0” instead of to the number of smaller absolute value since Math.floor()
returns the largest integer less than or equal to the given number.
Examples
An application of math.floor: How to Create a JavaScript Slot Machine
For this exercise, we have to generate three random numbers using a specific formula and not the general one. Math.floor(Math.random() * (3 - 1 + 1)) + 1;
Another example: Finding the remainder
Example
Usage
In mathematics, a number can be checked even or odd by checking the remainder of the division of the number by 2.
Note Do not confuse it with modulus%
does not work well with negative numbers.
More math-related articles:
jSlots is 2k of jQuery slot machine magic. It turns any list (<ol>
or <ul>
) into a slot machine!
Options
These are the options, with their default values, and what they do
Usage
Attach jQuery (successfully tested down to v1.4.1) /borderlands-presequel-slot-machine.html.
Attach jSlots plugin
Attach easing plugin (optional but HIGHLY recommended for nice animation)
Create a list and an element that will spin the slots
Target the list and make it a jSlot!
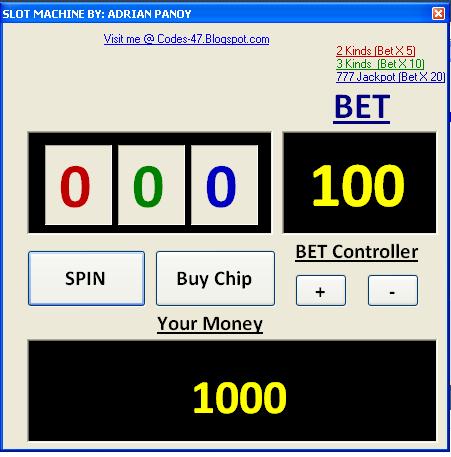
Styling is up to you, but jSlots supplies a jSlots-wrapper div around your lists that should get overflow: hidden
and a height set on it. Here are some recommended styles:
Published